Today, we’re going to take a look at how Red Hat tackles the creation of Microservices with Quarkus, a tool that not only facilitates agile development, but also supports business growth. Quarkus stands out on account of its ability to generate microservices with minimum weight and memory consumption. It’s specifically designed for deployment in container, serverless, cloud, and Kubernetes environments.
In previous posts, we’ve addressed Red Hat integrations, specifically Red Hat Fuse, now known as Red Hat Integration, which you can check out here. This Red Hat solution focuses on integrating systems with an up-to-date, cloud-oriented approach, commonly known as ESBs (Enterprise Service Bus) in legacy systems.
What is Red Hat Quarkus?
Quarkus is a lightweight Java framework, designed specifically for creating and deploying cloud-native applications, with minimal memory consumption. It’s tailored to comply with Jakarta EE specifications and relies on the MicroProfile, RESTEasy or Hibernate frameworks for microservices creation.
All of this is of course underpinned by the support of Red Hat, a multinational company that provides open source software solutions to enterprises. It’s seamlessly integrated into an industry-leading ecosystem of applications, including Kubernetes, ActiveMQ, Kafka, OpenShift, etc.
How to create Microservices with persistence with Quarkus
In this section, we outline a Red Hat tutorial that walks you through the creation of microservices with Quarkus step by step, from development to final deployment.
1- Development
When starting from scratch, it is best to create the structure of the project with the help of an archetype. This can also be carried out using one of the tools provided by Red Hat. In this instance, the quarkus-maven-plugin plugin and the following command:
mvn com.redhat.quarkus.platform:quarkus-maven-plugin:2.13.8.Final-redhat-00004:create. -DprojectGroupId=com.chakray.example -DprojectArtifactId=ms-with-panache
In order not to render it too basic, we’ll add persistence to the microservice. This will enable us to initiate a more complex microservice that will allow us to query, create and edit data from a database. In this case, we’ll make use of the following main libraries:
- REST API creation: quarkus-resteasy-reactive
- Data transformation and access: quarkus-hibernate-orm-panache
- Message formatting: quarkus-resteasy-reactive-jackson
The generation of the API that enables the microservice to provide an interface for third party systems can be one of the most straightforward steps, particularly for those with previous Java enterprise framework experience. Nevertheless, we’ve provided a brief explanation of how to generate any request method:
- @Path(“/book”): Allows us to specify the root of the microservice and the context in which it attends calls. It’s also used for each of the resources, should they have a different subsequent context.
- @GET, @DELETE, etc.: Indicates the HTTP verb with which the invocation will be performed.
- @QueryParam: Allows us to specify parameters that travel in the URL.
- @PathParam: Allows us to specify parameters that are part of the context. They must be specified in the @Path annotation.
- @Produces and @Consumes: Allows us to indicate the format of the output and the expected input.
We will use Panache for the persistence component, which will provide us with two different implementation patterns.
On the one hand we will have the Repository pattern, where we will create an intermediate class responsible for carrying out database operations. By extending the PanacheRepository class, we’ll have a wide set of basic access methods at our disposal.
@ApplicationScoped @Transactional public class BookRepository implements PanacheRepository<Book>{ }
In addition, we have the Active Record pattern, where the entity itself will provide the programmer with the various persistence methods.
@Entity @Table(name = "BOARDGAME") public class BoardGame extends PanacheEntity { //Careful! With PanacheEntity we don't need neither getter/setter nor id public String designer; public String name; }
This is our microservice’s main class:
@Path("/boardgame") public class BoardGameResource { @GET @Path("/{id}") @Produces(MediaType.APPLICATION_JSON) public BoardGame getBoardGameById(@PathParam("id") final Long id) { return BoardGame.findById(id); } @POST @Produces(MediaType.APPLICATION_JSON) @Consumes(MediaType.APPLICATION_JSON) @Transactional public BoardGame persist(final BoardGame newBoardGame) { BoardGame.persist(newBoardGame); return newBoardGame; } }
2- Configuration of a microservice with Quarkus
It’s possible to configure the various characteristics via the application.properties file. This is the only configuration file. Through this we are able to configure the libraries to be used within the project, in addition to specifying values for environments. Here are a few examples:
- http.port: We can specify the port for receiving calls.
- datasource.username/password/jdbc.url: We can specify the database connection.
- hibernate-orm: There are multiple options for specifying whether or not we want the database map to be generated upon application launch and the files for said purpose.
3- Deployment
Now that we’ve developed and configured the project, we’ll now proceed to launch it. As we’re still in the development phase, we’ll use the command:
mvn quarkus:dev
Another advantage of using the Red Hat Quarkus microservice is the fact that we can easily reload the application and verify changes by simply pressing the ‘s’ key on the console on which it is running.
And of course, we can generate native images to be used by Kubernetes through further configuration and Quarkus extensions that enable us to do so.
Conclusion
In summary, with the support of Red Hat and Chakray, your business can benefit from microservices that help drive growth. You’ll benefit from a technology that’s gaining momentum, generating agile, lightweight and cloud-oriented projects.
If you need help or support with Red Hat, we’re here to support your company. Contact us today.
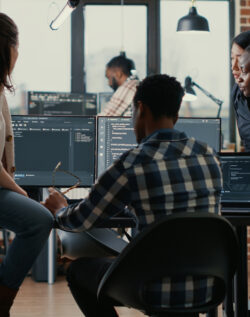
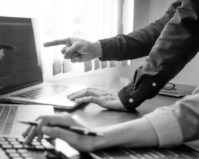
Talk to our experts!
Contact our team and discover the cutting-edge technologies that will empower your business.
contact us